An idle project after learning a bit about JavaScript
Boring playing T-rex game when your wifi is trouble again? Wanna make it more boring? Let's try make one!
Prepare The Tools
There are several tools that you will need:
1. VS Code
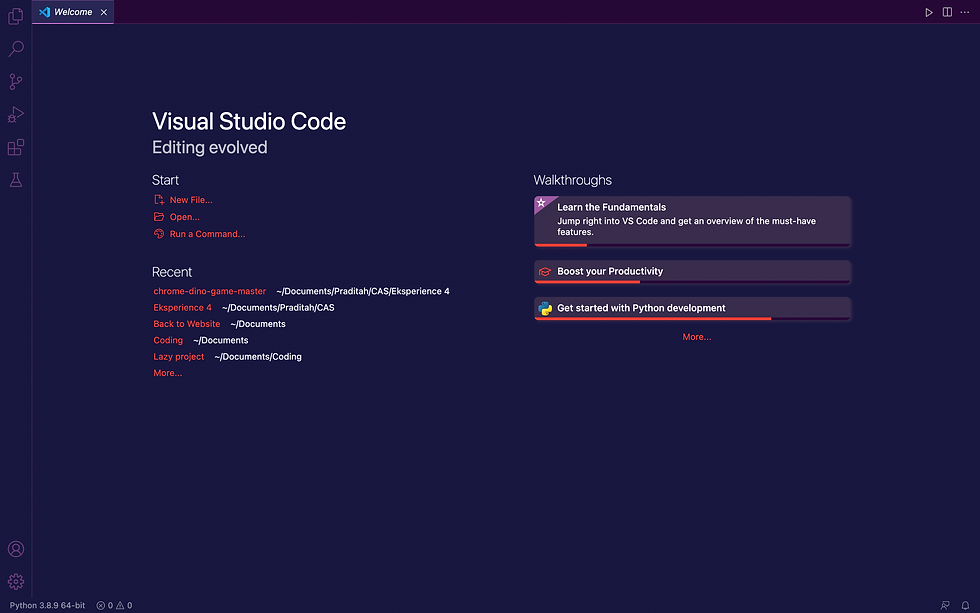
You can download it from the Visual Studio Code Website
2. This Dino
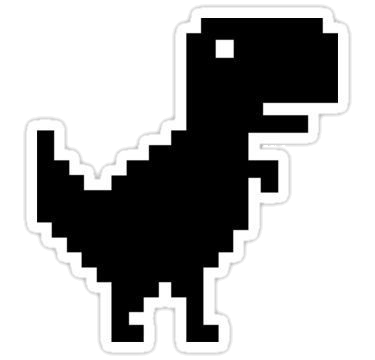
3. This Rock
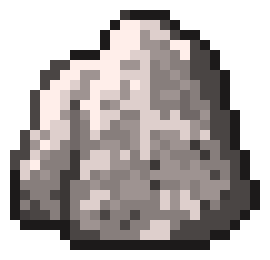
4. This Background
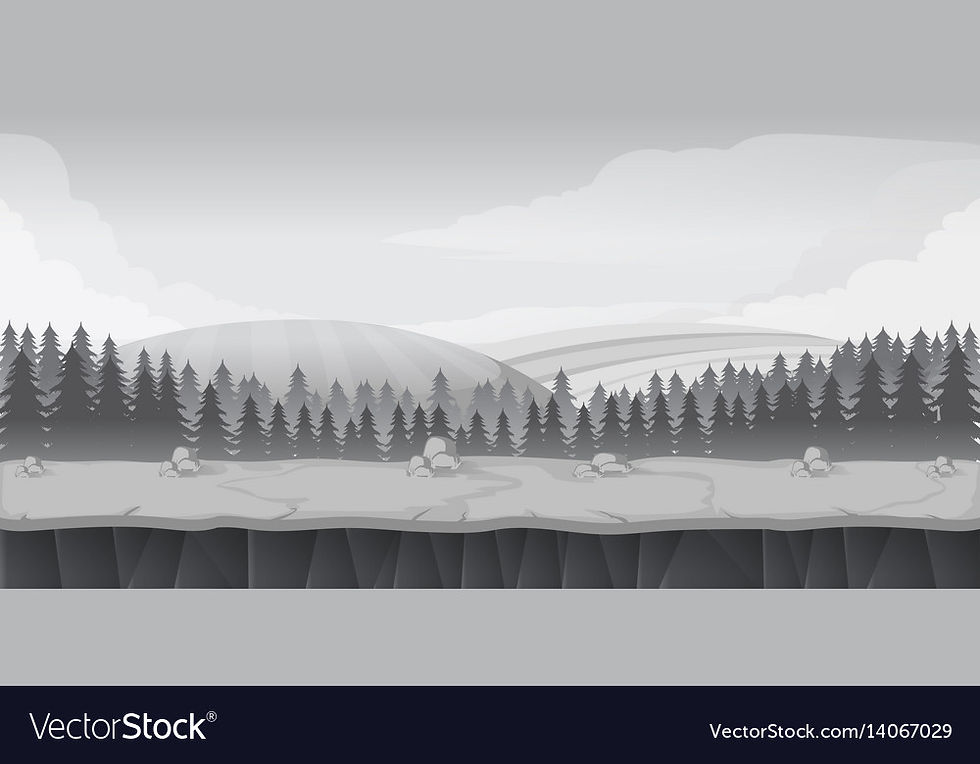
Make The Frame using HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Dino Game</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div id="game">
<div id="dino"></div>
<div id="rock"></div>
</div>
<h1 id="score">0</h1>
<script src="script.js"></script>
</body>
</html>
Make The Decoration using CSS
#score { text-align: center; }
#game {
width: 600px;
height: 300px;
border: 2px solid black;
margin: auto;
background-image: url("./background.jpg");
background-size: cover;
}
#dino {
height: 75px;
width: 75px;
top: 225px;
position: relative;
background-image: url("./dino.png");
background-size: cover;
}
#rock {
width: 50px;
height: 50px;
position: relative;
top: 175px;
left: 550px;
background-image: url("./rock.png");
background-size: cover;
animation: rock 1.33s infinite;
}
@keyframes rock {
0%{left: 550px;}
100%{left: -50px;}
}
.jump-animation {
animation: jump 0.5s;
}
@keyframes jump {
0%{top: 225px;}
50%{top: 75px;}
75%{top: 75px;}
100%{top: 225px;}
}
Make The Movement using Javascript
const dino = document.getElementById("dino");
const rock = document.getElementById("rock");
const score = document.getElementById("score");
function jump() {
dino.classList.add("jump-animation");
setTimeout(() =>
dino.classList.remove("jump-animation"), 500);
}
document.addEventListener('keypress', (event) => {
if (!dino.classList.contains('jump-animation')) {
jump();
}
})
setInterval(() => {
const dinoTop = parseInt(window.getComputedStyle(dino)
.getPropertyValue('top'));
const rockLeft = parseInt(window.getComputedStyle(rock)
.getPropertyValue('left'));
score.innerText++;
if (rockLeft < 0) {
rock.style.display = 'none';
} else {
rock.style.display = ''
}
if (rockLeft < 50 && rockLeft > 0 && dinoTop > 150) {
alert("You got a score of: " + score.innerText +
"\n\nPlay again?");
location.reload();
}
}, 50);
So We Just Copy-Paste It?
HTML
No, let just analyze it one by one
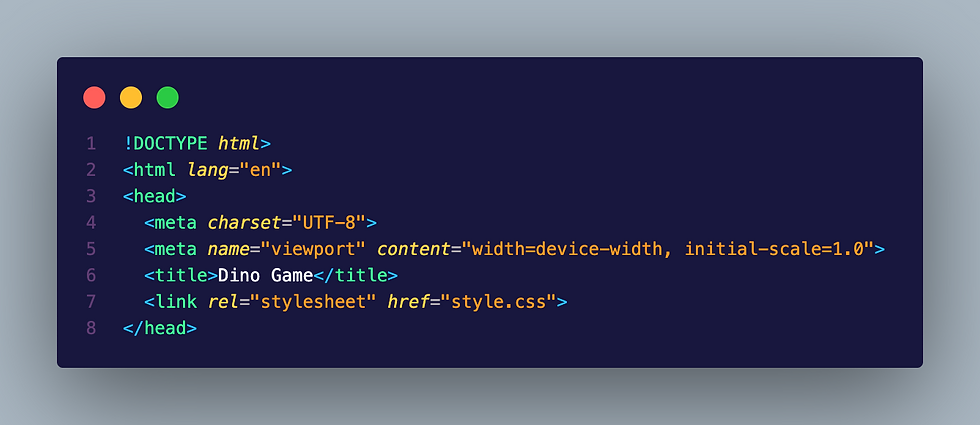
It is the meta-data of HTML, just a standard to initiate our HTML code and usually are generated from embed snippet. If you use VSCode, you could press ! + tab to automatically create the those meta-data and also body framework. However there are some exception,
<title> Dino Game </title> is to name the tab when it is opened in the browser. You could see in the top of your screen, when you opened wikipedia or anything, the name of the tab is based on the text inside the <title> tag. If you bored with Dino Game, you could change the name to anything that you want as long as you write it inside the tag.
<link rel="stylesheet" href="style.css"> is to import stylesheet (Style command to decorate the HTML using css) from file style.css which will we make next. It is also changeable depend on what file you want to reference.
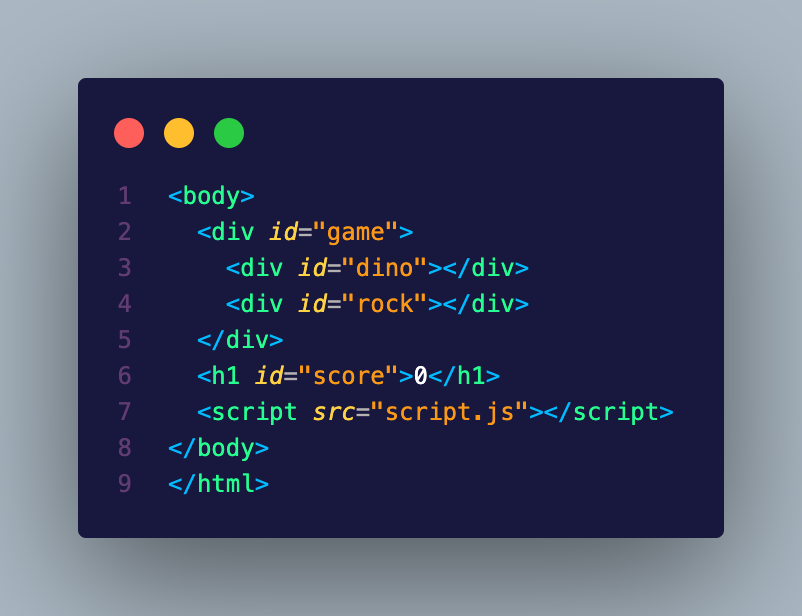
It is the body of the html. In HTML there are 2 parts: <head> which is contain meta-data that not appear on the screen and <body> where the content that we want to display contained.
<body> to wrap all of the content that you want to display
<div> to wrap a content into a part inside the body. Id is optional but recommended. id is act as the name-tag of a div, Thus it will be easier to make an individual style from CSS and javascript for the div with specified id.
I make div "game" to wrap "dino" and "rock" div. As the name, both tag will contain moving dino and rock that appear like in the first image above.
<h1> means heading 1, it is display text. You can also embed id in this tag. I use id score to display the score later
<script> is to import the javascript command from javascript, in this case: script.js in the same folder which we will make next. I dunno why some reference use href while the other use src.
CSS
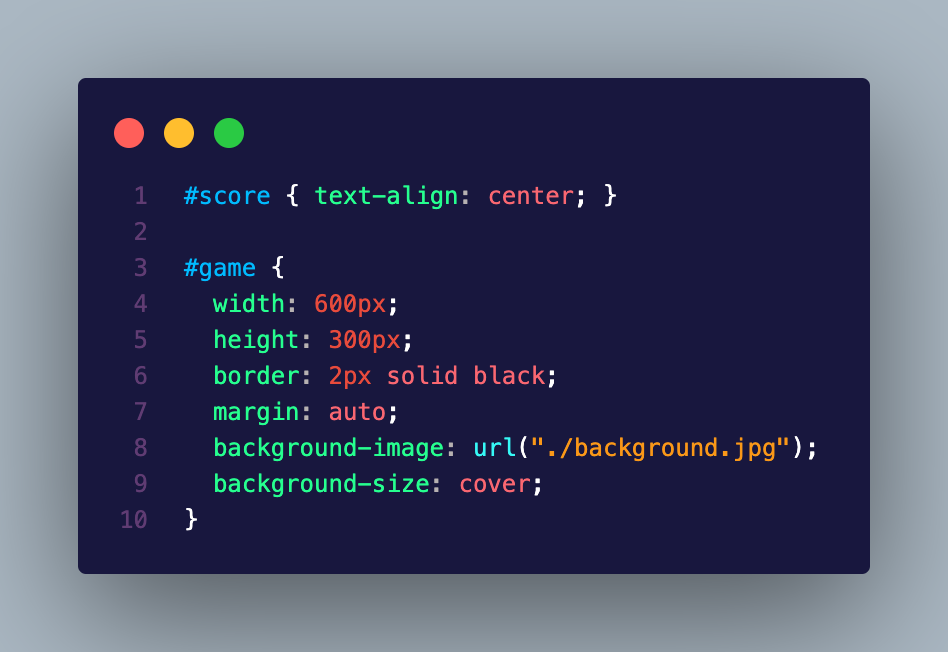
# sign is to make a style for id in this case #score is for decorate id score and #game for id game.
text-align is used to aligning the text, instead of center there are another option such as left, right, and justify, you could see the reference
Width is to set the width of the div and height for the height.
The border is set to have weight of 2px or you can change it from 0 to infinity as long as it is logic. Then solid is to styling the border as normal border. You can also change it as dashed, dotted, etc, you can see the reference. Then the last line for the color of the border. The input could be the name of color - however it is not favorable since the option is limited - or i usually use hex color. Thus you can see the pattern:
border: size style color;
Background image is to attach an image to fill the div with image.
Background size is to styling the background image. Cover means resize the background image to cover the entire container, even if it has to stretch the image or cut a little bit off one of the edges.

Then we will make the style for the dino. The dino will be placed above the game div since the div code in HTML is written inside the game div. There are 2 new tag in our css: top and position.
Top is a property that affects the vertical position of a positioned element. This property has no effect on non-positioned elements. The position is based written in the position tag. Position tag is to posit the div in the screen.
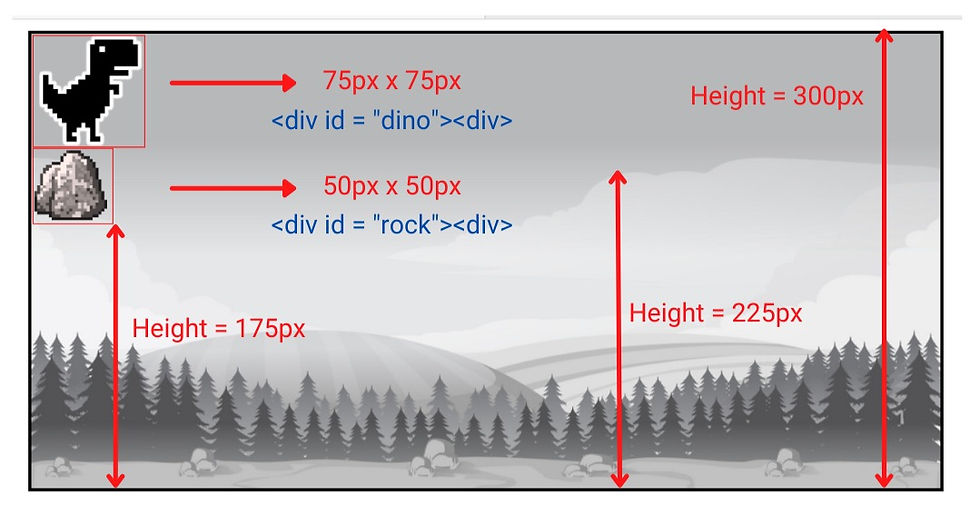
An element with position: relative; is positioned relative to its normal position. Setting the top, right, bottom, and left properties of a relatively-positioned element will cause it to be adjusted away from its normal position. Other content will not be adjusted to fit into any gap left by the element.
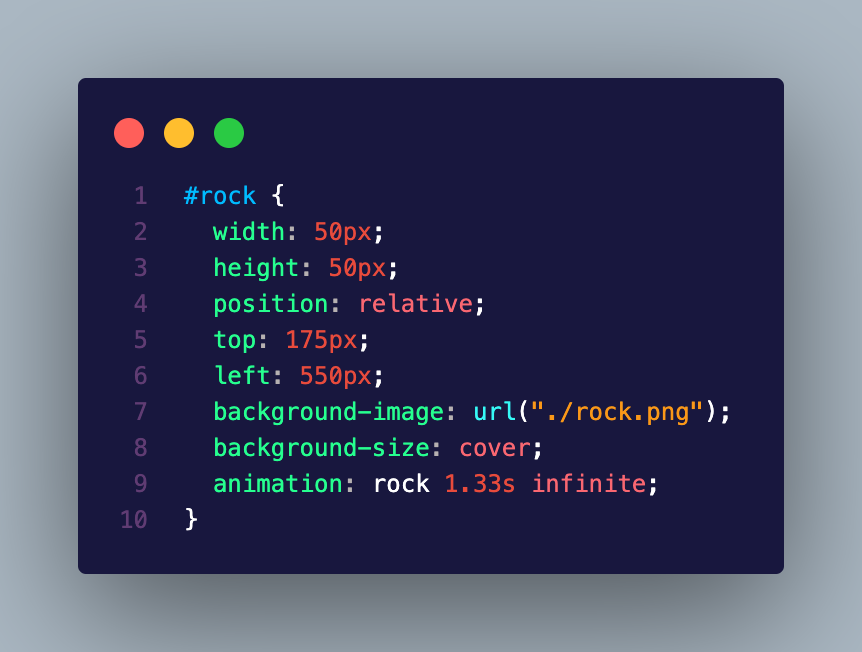
Now for the position will be a little complicated but i already insert an image to explain it above.
New again, yes it is animation world. Using CSS you can make a thing in HTML - as example our rock div - moving. You can see more in animation reference.
So we use "rock" animation with duration of 1.33 s and it looped forever (infinite). Rock is our custom animation that we declare below this line.
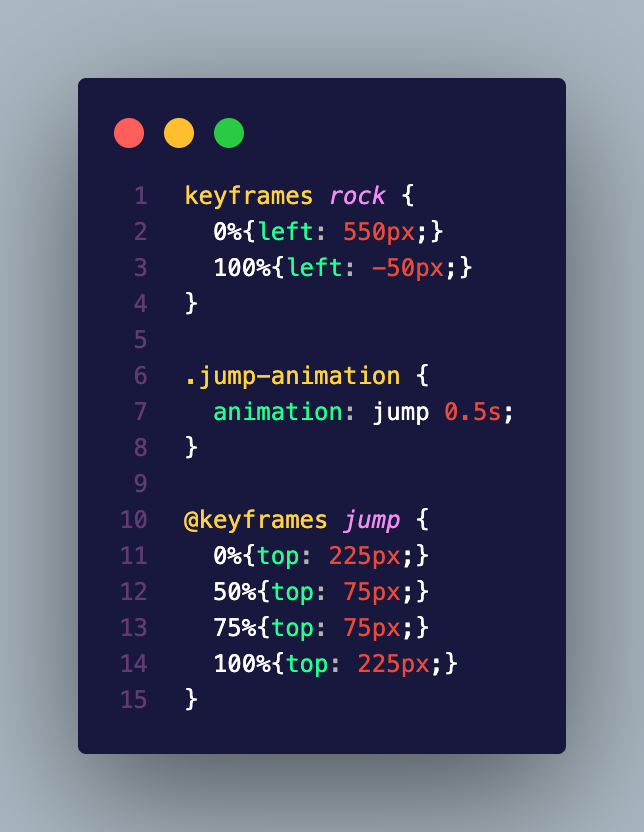
My fault, i forgot to put @ in front of keyframe rock, you should add it by yourself
The keyframes told us that the movement of the rock is from position (left: 550px) to the maximum (left: -50px). Then we combine with the previous animation properties on #rock thus the duration will become 1.3 s and it will loop forever.
.jump-animation is to styling class jump-animation. . is an identifier that this style is belong to class, class is another level of HTML besides id that could be use in more than one object, while id is only for one object. The duration of "jump" animation is 0.5s.
Then jump animation defined as vertical movement of from jumping from 225px to 75px in 50% of time which mean 0,25s then hover for 0,125s and go down again for 0.125s.
Javascript
I hope your brain doesn't blow yet to understand this simple thing because we will enter the hardest thing in this system.

Okay so javascript didn't know what the hell is div with dino nor rock nor score. Well actually they know it but if you want to call them you should write:
document.getElementById("blablabla")
And it is annoying, it is a long code, and ineffective. Thus we make a shortcut, we wrap the div name to something that javascript could understand. A variable that namely as the same as the div id was. Thus, we could call them without using those long code. Actually there are 4 types of variable there are const, var, let, or nothing, you could see more on the attached link.
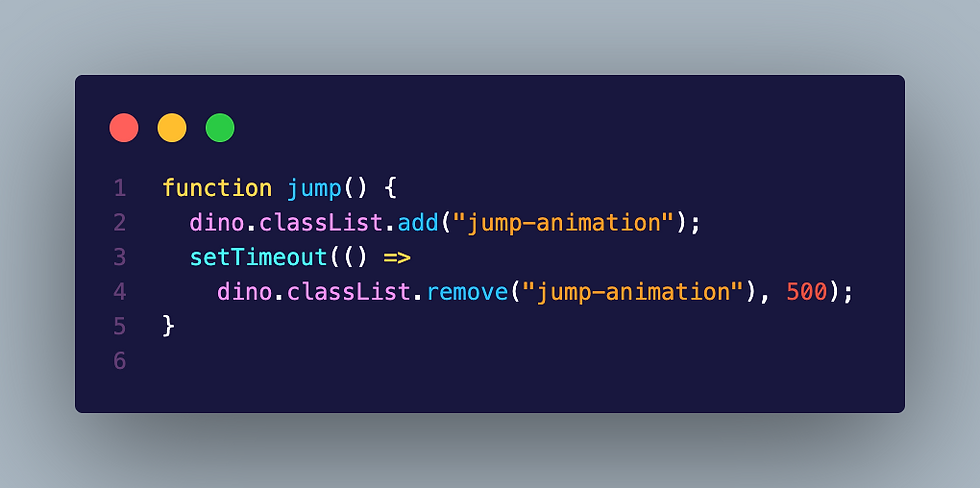
Well in this case we will make a function of jump to become accessible in javascript. Using this code we could add jump-animation to the desired action. Later we will attached this code in someplace so when we press space button - actually any button except power - the jump function will be executed.
dino.classList.add("jump-animation") -> to insert jump-animation inside function jump which will execute jump-animation in the dino variable in javascript that connected with dino div in HTML.
setTimeout -> to set a time for 500 ms (0.5 s) which the action will be stopped after that time by make a command dino.classList.remove("jump-animation")
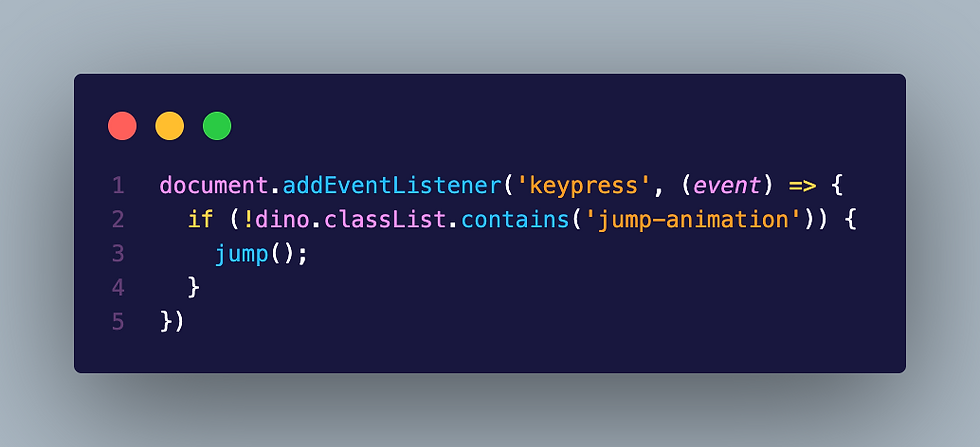
Using this code we could press any button in our keyboard to call the jump() function which we already declared before. We could see the pattern:
element.addEventListener(event function)
Keypress -> event, we could replace it with "click", "mouseover", etc.
(event) -> this is a function actually which check the condition when the code is running whether the dino.classList contains "jump-animation" or not. If it is not true then the jump() will not be executed and if false jump() will be executed. Beware with ! sign in the beginning of the code, it indicate inverse condition. You can proof by double jumping, it would not happen!
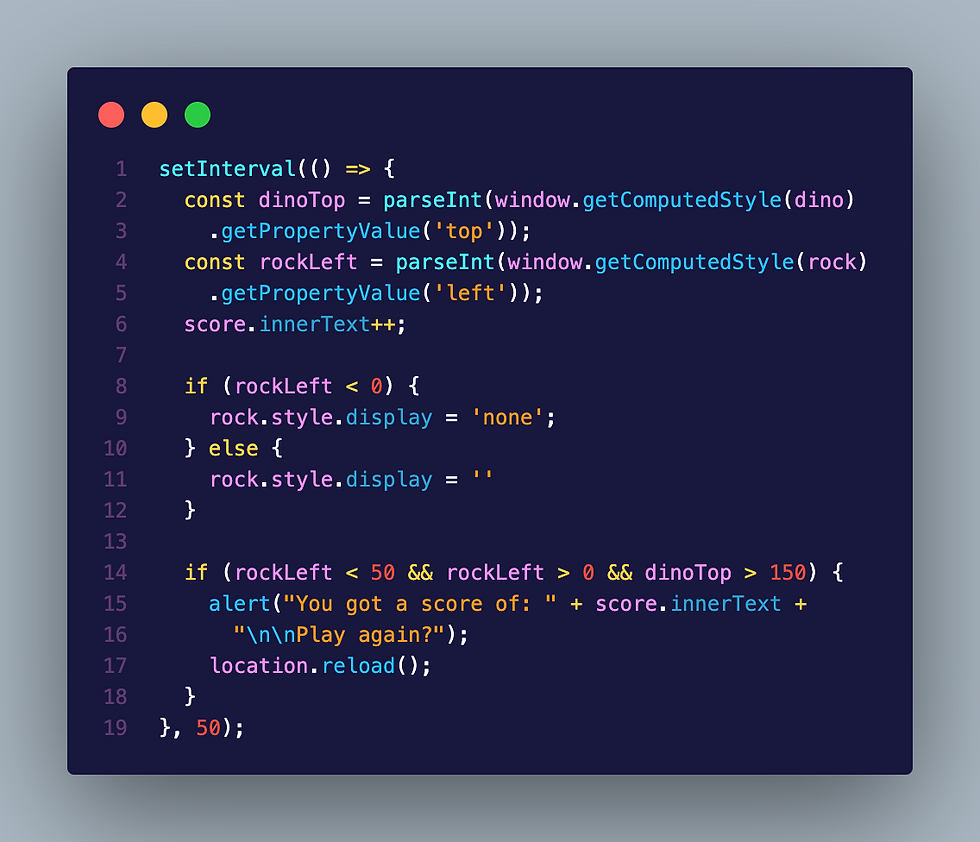
Well it is long, but it is the last.
Different from setTimeout() which executed once, The setInterval() method continues calling the function until clearInterval() is called, or the window is closed.
Weird code in line 2-3 is to import the real-time value of 'top' properties from the dino's CSS into variable namely dinoTop. You know it change due to jumping, isn't it? While the 4-5 line is to import left properties from the rock. Remember, the rock move from right to left.
score.innerText++ is to increment the value of score so it is always add by one in every 50 ms.
if statement in line 8-12 is to loop the rock so it always appear every whenever it hit left = 0 or the left end of the background
The last code is to state that if the position of the dino is below 150px and the rock is in the position that possible to hit the dino which is 0px < x < 50px, that mean the dino is hit the rock and game over. The alert() will give an alert that display your score and a button to play again by connecting it with location.reload which means reloads the current document.
Comments